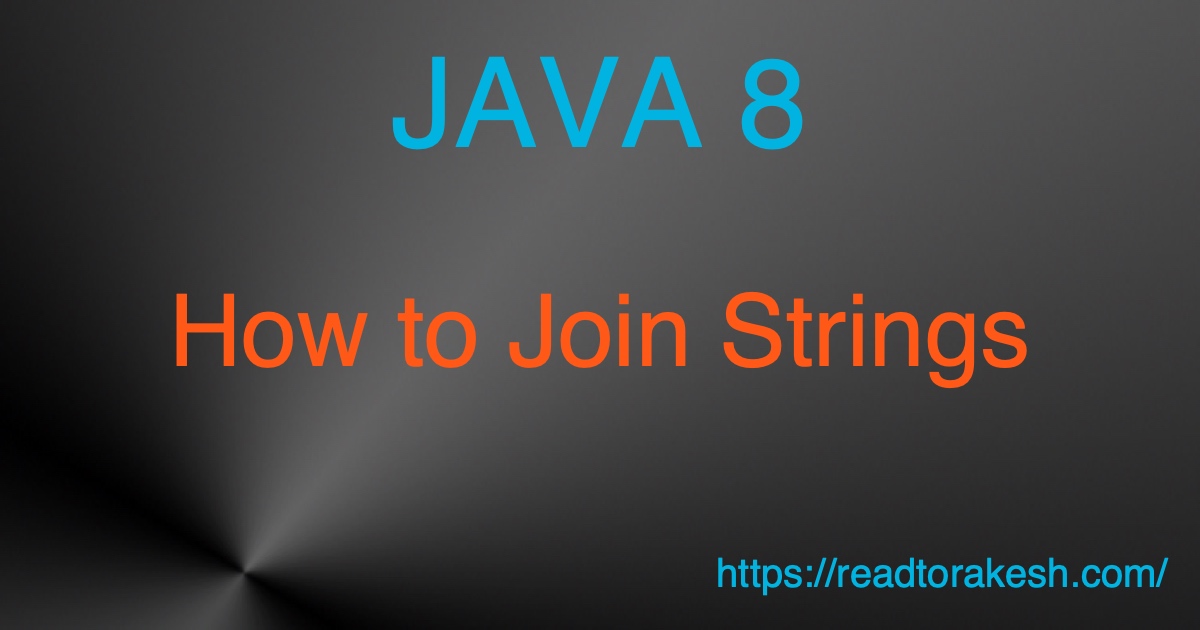
As a programmer quite often we hit the need to join multiple string values separated by a delimiter. For example join all values from a String[] or List separated by comma ( , ). It’s very common scenario and I am sure most of have already done this. Lets how it’s usually done before Java 8 and what is smart way to Join multiple strings in Java 8.
We have following array of strings and we need to join all string values from this array into single string separated by pipe ( | ) character
String[] quote = {"Java8", "is", "awesome"};
Before Java 8
Before Java 8 usual way to do is, loop through each element of the array and append to another String variable and append separator after each element. May be you are already thinking about general issue with this approach which is unnecessary separator after the last element. To deal with it, either we can add condition within loop to not append separator after last element or remove the last character (the extra separator) later on after the loop. Lets go with first option. The code will look like below.
String[] quote = {"Java8", "is", "awesome"};
StringBuffer combined = new StringBuffer();
for(int i=0;i<quote.length;i++) {
combined.append(quote[i]);
if(i < quote.length - 1) {
combined.append("|");
}
}
System.out.println("Output: " + combined.toString());
--- Output ---
Output: Java8|is|awesome
You can notice the annoyance of extra separator issue. Even for such a simple task of joining strings, we had to write logic which of course comes with overhead of unit testing.
In Java 8
In Java 8 this simple task remains simple even in the code. In Java 8 String class has static utility function calls join(…) which makes it very easy to join multiple strings. join function tables 2 parameters. First parameter is delimiter and second parameter is array of strings to join.
String[] quote = {"Java8", "is", "awesome"};
String combined = String.join("|", quote);
System.out.println("Output: " + combined);
--- Output ---
Output: Java8|is|awesome
Actual code to join strings is just 1 line and very readable. No logic, no loop, no condition. Simple task look real simple code and generates exactly same output.
join method is overloaded with another flavor which takes second parameter as List<String>
List quote = Arrays.asList(new String[] {"Java8", "is", "awesome"});
String combined = String.join("|", quote);
System.out.println("Output: " + combined);
--- Output ---
Output: Java8|is|awesome
Under the hood String.join(…) uses java.util.StringJoiner which is new addition in Java 8. We can directly use StringJoiner class as shown below.
List quote = Arrays.asList(new String[] {"Java8", "is", "awesome"});
StringJoiner stringJoiner = new StringJoiner("|");
for(String item: quote) {
stringJoiner.add(item);
}
String combined = stringJoiner.toString();
System.out.println("Output: " + combined);
--- Output ---
Output: Java8|is|awesome
StringJoiner constructor takes joining separator as parameter. You can adding multiple strings to join by calling add method and toString method gives combined string.
StringJoiner has overloaded constructor. Another flavor of constructor takes Prefix and Postfix to be used in joining operation. See below
List quote = Arrays.asList(new String[] {"Java8", "is", "awesome"});
StringJoiner stringJoiner = new StringJoiner("|", "[", "]");
for(String item: quote) {
stringJoiner.add(item);
}
String combined = stringJoiner.toString();
System.out.println("Output: " + combined);
--- Output ---
Output: [Java8|is|awesome]
Please share it and help others if you found this blog helpful. Feedback, questions and comments are always welcome.